Securing Your React Applications: Authentication and Authorization
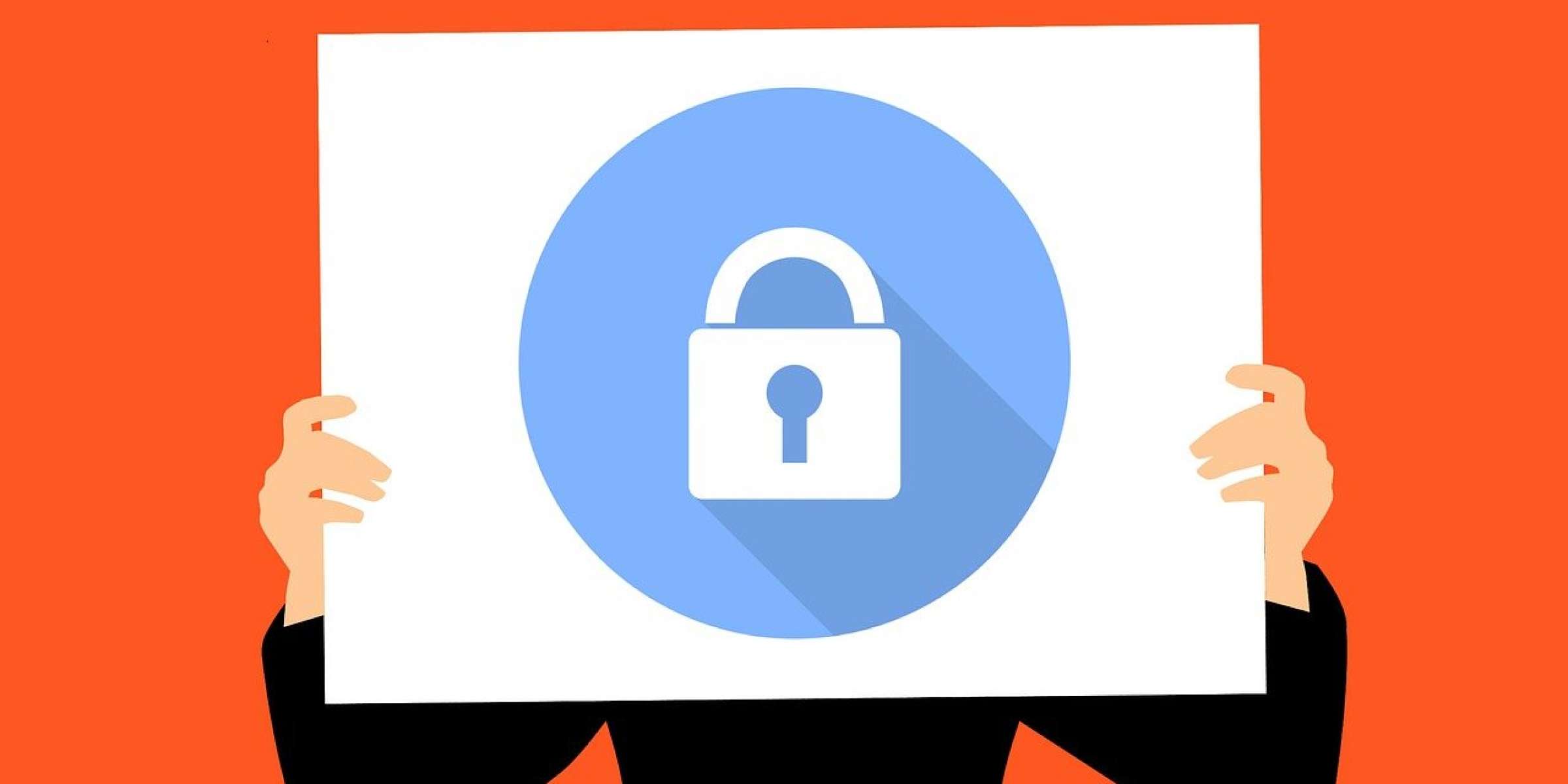
In today's digital landscape, security is paramount, especially when it comes to web applications. Ensuring that your React applications are properly secured with robust authentication and authorization mechanisms is essential to protect sensitive user data and maintain the integrity of your application. In this blog, we'll delve into the fundamentals of authentication and authorization in React applications, highlighting key concepts and best practices to keep your apps secure.
Understanding Authentication and Authorization
What is Authentication?
Authentication is the process of verifying the identity of a user attempting to access a system or application. It ensures that the user is who they claim to be before granting access to protected resources. In the context of web applications, authentication typically involves validating user credentials, such as usernames and passwords.
What is Authorization?
Authorization, on the other hand, determines what actions an authenticated user is allowed to perform within the application. It involves defining and enforcing access control rules based on the user's identity and assigned permissions. Authorization ensures that users only have access to the resources and functionalities that they are authorized to use.
Implementing Authentication in React Applications
1. User Authentication Workflow
Highlight:
- User enters credentials (username/password).
- Credentials are sent to the server for validation.
- If valid, the server generates a token (e.g., JWT).
- The token is sent back to the client and stored securely (e.g., local storage, cookies).
2. Using JWT for Token-Based Authentication
Highlight:
- JWT (JSON Web Tokens) are compact, URL-safe tokens that can be securely transmitted between parties.
- They consist of three parts: header, payload, and signature.
- JWTs can store user identity and additional claims, such as expiration time and permissions.
3. Securely Storing and Managing Tokens
Highlight:
- Tokens should be stored securely to prevent unauthorized access.
- Consider using browser storage mechanisms like localStorage or sessionStorage.
- Implement mechanisms to mitigate common security threats, such as XSS (Cross-Site Scripting) attacks.
Implementing Authorization in React Applications
1. Role-Based Access Control (RBAC)
Highlight:
- RBAC assigns roles to users and defines permissions based on those roles.
- Roles can include privileges such as admin, user, moderator, etc.
- Implement authorization middleware to check if the user's role allows access to specific resources.
2. Fine-Grained Access Control
Highlight:
- Define granular access control policies for individual resources or actions.
- Utilize conditional rendering in React components to display or hide UI elements based on the user's permissions.
- Regularly review and update access control policies to adapt to changing requirements or security threats.
Best Practices for Secure React Applications
1. Use HTTPS for Secure Communication
Highlight:
- Ensure that all communication between the client and server is encrypted using HTTPS.
- HTTPS prevents eavesdropping and man-in-the-middle attacks, ensuring the confidentiality and integrity of data.
2. Keep Dependencies Updated
Highlight:
- Regularly update dependencies, including React, libraries, and frameworks, to patch security vulnerabilities.
- Use tools like npm audit to identify and fix security issues in your project dependencies.
Conclusion
Securing your React applications requires a multi-layered approach, encompassing both authentication and authorization mechanisms. By implementing robust authentication protocols, securely managing user tokens, and enforcing fine-grained access control, you can mitigate security risks and safeguard your application against unauthorized access and data breaches. Remember to stay informed about the latest security best practices and continuously monitor and update your security measures to stay one step ahead of potential threats.
Consult us for free?
View More